The longValue()
method of Integer class, returns the value represented by this Integer object as long. Since the size of long is greater than the size of int data type, It is also called widening primitive conversion.
Syntax of longValue() method
public long longValue()
longValue() Parameters
- It does not have any parameter.
longValue() ReturnValue
- Returns a long value. This value is numerically equivalent to the value contained in this Integer object.
Example 1
This example demonstrates the use of longValue()
method. Here, we have an Integer object and we are converting the value of it into a long primitive data type.
class JavaExample { public static void main(String args[]) { Integer iObj = new Integer(400); long lNum = iObj.longValue(); //Integer to long System.out.println("Integer object value: "+iObj); System.out.println("long value: "+lNum); } }
Output:
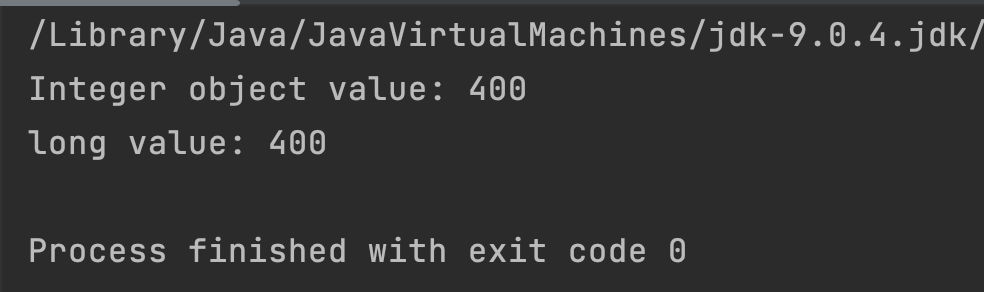
Example 2
Given string contains an integer number. This string is passed to the constructor of Integer class to convert this string into an Integer object. The Integer object then converted into a long value using longValue()
method.
class JavaExample { public static void main(String args[]) { String str = "45678"; Integer iObj = new Integer(str); //String to Integer long lNum = iObj.longValue(); //Integer to long System.out.println("Integer object value: "+iObj); System.out.println("long value: "+lNum); } }
Output:
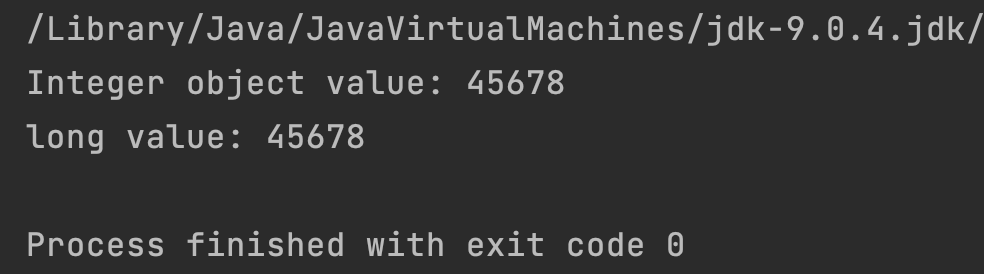