The min() method of Integer class, returns smaller of two int numbers passed as arguments to this method. It works similar to Math.min() method.
Hierarchy:
java.lang Package -> Integer Class -> min() Method
Syntax of min() method
public static int min(int a, int b)
min() Parameters
a
– First operand.b
– Second operand.
min() Return Value
- Returns smaller number between the two int arguments
a
andb
.
Supported versions: Java 1.8 and onwards.
Example 1
public class JavaExample { public static void main(String[] args) { int a = 15, b = 6; System.out.println("Given numbers are: "+a+", "+b); System.out.println("Smaller of given numbers: "+Integer.min(a,b)); } }
Output:

Example 2
public class JavaExample { public static void main(String[] args) { int a = -15, b = -6; System.out.println("Given negative numbers are: "+a+", "+b); System.out.println("Smaller of given numbers: "+Integer.min(a,b)); } }
Output:
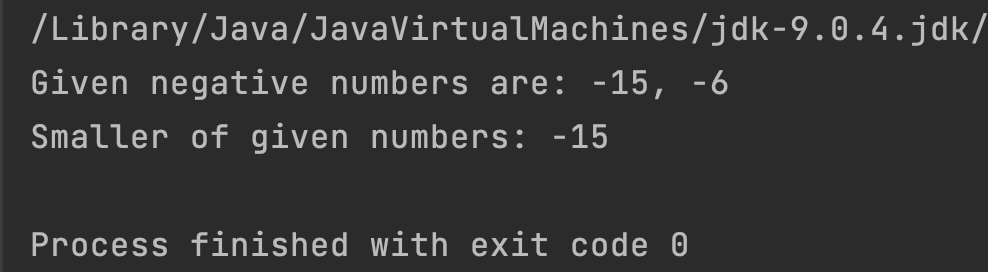
Example 3
If one of the given number is Integer.MIN_VALUE, then min() method always returns Integer.MIN_VALUE as result.
public class JavaExample { public static void main(String[] args) { int a = -15, b = Integer.MIN_VALUE; System.out.println("Smaller of given numbers: "+Integer.min(a,b)); System.out.println(Integer.MIN_VALUE); } }
Output:
