Java StringBuffer class is used to create mutable strings. A mutable string is the one which can be modified. StringBuffer is an alternative to Java String class. In this guide, we will discuss StringBuffer class in detail. We will also cover important methods of Java StringBuffer class with examples.
Constructors of StringBuffer class
Constructor | Description |
StringBuffer() | This is the default constructor of the StringBuffer class, it creates an empty StringBuffer instance with the default capacity of 16. |
StringBuffer(String str) | This creates a StringBuffer instance with the specified string. |
StringBuffer(int capacity) | This creates StringBuffer instance with the specified capacity instead of the default capacity 16. |
StringBuffer(CharSequence cs) | It creates a StringBuffer instance with the specified character sequence. |
Example of various constructors of StringBuffer class:
public class JavaExample { public static void main(String args[]) { //creating StringBuffer object using default constructor StringBuffer sb = new StringBuffer(); //appending a string to newly created instance sb.append("BeginnersBook.com"); System.out.println("First String: "+sb); //Using StringBuffer(String str) constructor String str = "hello"; StringBuffer sb2 = new StringBuffer(str); System.out.println("Second String: "+sb2); //Using StringBuffer(int Capacity) constructor StringBuffer sb3 = new StringBuffer(24); System.out.println("Capacity of sb3: "+sb3.capacity()); //creating StringBuffer(CharSequence cs) constructor StringBuffer sb4 = new StringBuffer("Welcome"); System.out.println("Fourth String: "+sb4); } }
Output:
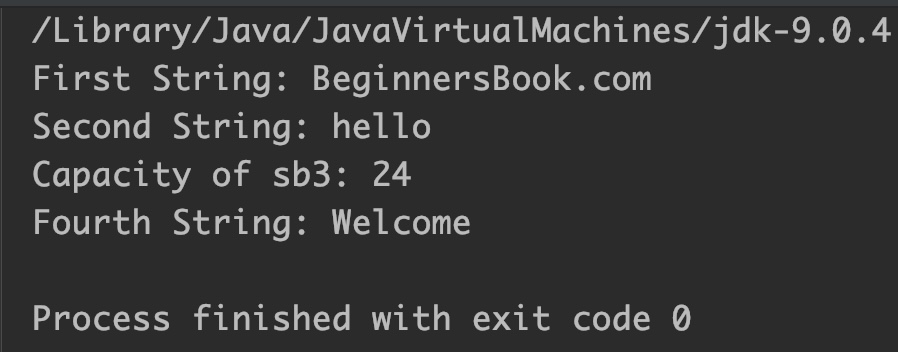
Methods of StringBuffer class in Java
Method Signature | Description |
public synchronized StringBuffer append (String str) | It appends (concatenates) the specified string str to the already existing StringBuffer value. |
public synchronized StringBuffer insert (int pos, String str) | It inserts the specified string str at the specified position pos . |
public synchronized StringBuffer replace(int startIndex, int endIndex, String str) | It replaces the string between the specified startIndex and endIndex with the specified string str . |
public synchronized StringBuffer delete(int startIndex, int endIndex) | It deletes a portion of the string from the specified startIndex till endIndex . |
public synchronized StringBuffer reverse() | It is used to reverse the given string. |
public int capacity() | It prints the current capacity of the StringBuffer instance |
public void ensureCapacity(int minElements) | It increases the capacity of the StringBuffer to ensure that it can hold at least the mentioned minElements . |
public char charAt(int index) | Returns the character |
public int length() | Returns the length of the current string. |
public String substring(int startIndex) | Returns substring starting from the specified startIndex . |
public String substring(int startIndex, int endIndex) | Returns substring starting from the specified startIndex till specified endIndex . |
public int indexOf(String subStr) | It returns the index of first occurrence of the specified substring subStr in the given string. |
public int lastIndexOf(String str) | It returns the index of the last occurrence of the specified substring subStr in the given string. |
public void trimToSize() | It attempts to trim the free size of the StringBuffer, if possible. |
public int codePointBefore() | It returns the unicode code point value for the character before the specified index. |
Java StringBuffer Examples
Example 1: StringBuffer insert() method
public class JavaExample { public static void main(String args[]) { StringBuffer sb = new StringBuffer("This is Text"); //insert "a Sample" string after "is" //index starts with 0 so the index of s is 6 sb.insert(7, " a Sample"); System.out.println(sb); } }
Output:
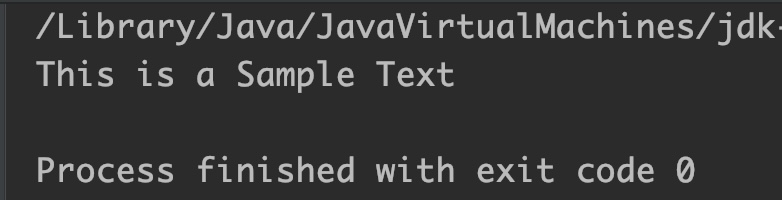
Example 2: StringBuffer append() method
public class JavaExample { public static void main(String args[]) { StringBuffer sb = new StringBuffer("Welcome to"); //appending specified string at the end of given string sb.append(" BeginnersBook.com"); System.out.println(sb); } }
Output:
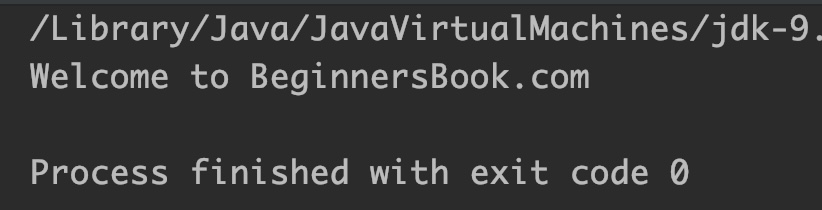
Example 3: StringBuffer replace() method
public class JavaExample{ public static void main(String args[]){ StringBuffer sb=new StringBuffer("Hi Guys!"); //replace "Hi" with "Hello" sb.replace(0,2,"Hello"); System.out.println(sb); } }
Output:
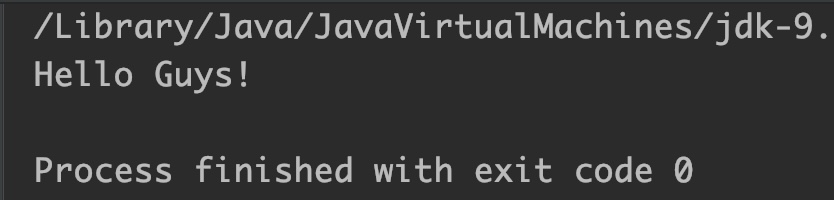
Example 4: StringBuffer reverse() method
public class JavaExample{ public static void main(String args[]){ StringBuffer sb=new StringBuffer("Text"); //reverses the string "Text" sb.reverse(); System.out.println("Reversed String: "+sb); } }
Output:
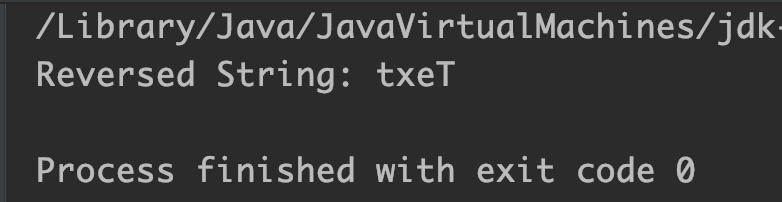
Example 5: StringBuffer delete() method
public class JavaExample{ public static void main(String args[]){ StringBuffer sb=new StringBuffer("BeginnersBook"); //delete substring "Beginners" sb.delete(0, 9); System.out.println(sb); } }
Output:
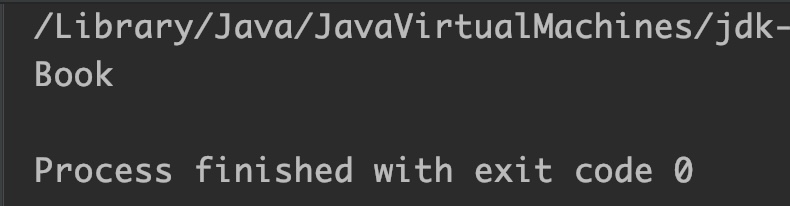
Example 6: StringBuffer capacity() method
public class JavaExample{ public static void main(String args[]){ StringBuffer sb=new StringBuffer(); //default capacity 16 System.out.println("Current Capacity: "+sb.capacity()); //appended string is 13 chars, can be adjusted in current //capacity so no capacity increase sb.append("BeginnersBook"); System.out.println("Current Capacity: "+sb.capacity()); //Need to increase capacity to append //this string. (16*2) +2 = 34 sb.append("Book"); System.out.println("Current Capacity: "+sb.capacity()); //34 } }
Output:
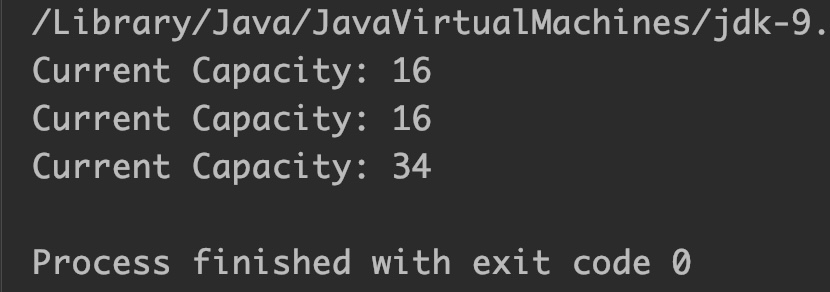
Example 7: StringBuffer length() method
public class JavaExample{ public static void main(String args[]){ StringBuffer sb=new StringBuffer(); sb.append("Welcome"); System.out.println("Length of string: "+sb.length()); } }
Output:
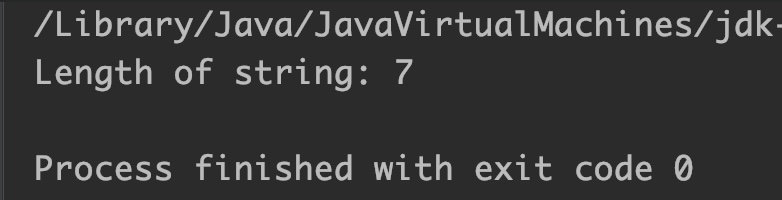
Example 8: StringBuffer indexOf() method
public class JavaExample{ public static void main(String args[]){ StringBuffer sb=new StringBuffer(); sb.append("Welcome"); System.out.println("String: "+sb); System.out.println("Index of substring come: "+sb.indexOf("come")); } }
Output:
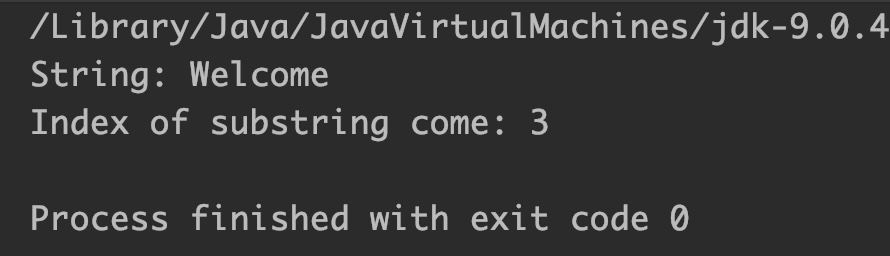
Example 9: StringBuffer charAt() method
public class JavaExample{ public static void main(String args[]){ StringBuffer sb=new StringBuffer(); sb.append("Welcome"); System.out.println("String: "+sb); System.out.println("Char at index 3 is: "+sb.charAt(3)); } }
Output:
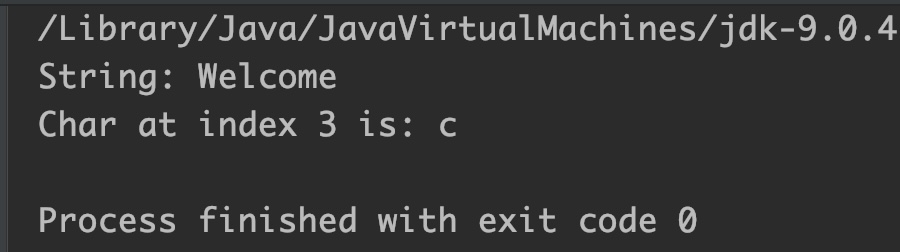
Conclusion
In this article, we discussed Java StringBuffer class and its methods with examples. To learn more on strings, refer Java String and Java StringBuilder tutorials. Also, you can head over to our Java tutorial section to start learning other such guides in detail.